Essential Java Coding Standards: Writing Code That Speaks Volumes
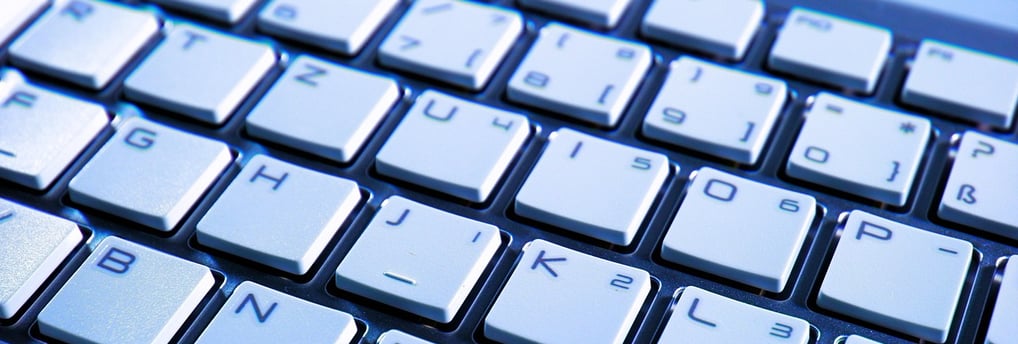
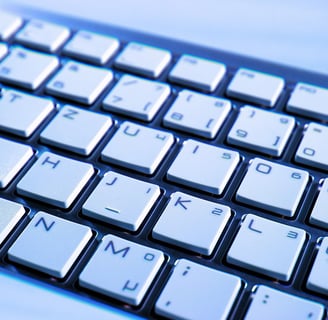
In the vast realm of programming languages, Java stands tall as one of the most popular and versatile choices for developers worldwide. Its flexibility and robustness make it ideal for a wide array of applications, from web development to mobile apps and enterprise-level software. However, with great power comes the responsibility of maintaining clean, readable, and maintainable code. This is where Java coding standards come into play.
Why Coding Standards Matter
Think of coding standards as the rules of the road for developers. They ensure consistency across a codebase, making it easier for team members to understand each other's code, collaborate effectively, and maintain the software over time. When everyone follows the same set of guidelines, the code becomes more predictable, less error-prone, and easier to debug.
Clarity and Readability
One of the primary objectives of coding standards is to enhance the readability of the code. When a developer looks at a piece of Java code, they should be able to quickly grasp its purpose and functionality. This clarity is achieved through consistent naming conventions, proper indentation, and well-defined code structure.
For instance, meaningful variable and method names can work wonders in making code self-documenting. Instead of using cryptic abbreviations or single-letter variables, opt for descriptive names that convey the intent. This not only helps fellow developers understand the code faster but also makes your code more comprehensible when you revisit it after some time.
Consistency Across the Board
Consistency is key to writing maintainable Java code. Imagine working on a project where each developer follows their formatting style, naming conventions, and code organization. Chaos ensues, making it a Herculean task to merge changes, debug issues, or onboard new team members.
Coding standards establish a unified style guide for the entire team to follow. Whether it's the placement of curly braces, the indentation level, or the use of whitespace, adhering to a consistent style across the codebase ensures that the project remains cohesive and manageable.
Bug Prevention and Code Quality
Well-defined coding standards can also act as a proactive measure against bugs and errors. By mandating best practices such as proper exception handling, avoidance of magic numbers, and consistent error checking, developers are less likely to introduce common pitfalls into the code.
Moreover, following coding standards often leads to improved code quality. When developers are encouraged to write clean, concise, and modular code, the overall quality of the software naturally rises. This not only benefits the current development cycle but also pays dividends in terms of easier maintenance and extensibility in the future.
Essential Java Coding Standards
Now that we understand the importance of coding standards, let's delve into some essential guidelines specifically tailored for Java development.
1. Naming Conventions
● Classes and Interfaces: Use nouns or noun phrases, starting with an uppercase letter. For example, Customer, AccountService.
● Methods: Use verbs or verb phrases, starting with a lowercase letter. For example, calculateTotal(), getUserById().
● Variables: Follow camelCase for variables, starting with a lowercase letter. For example, int itemCount, String userName.
2. Code Formatting
● Indentation: Use 4 spaces for each level of indentation. This enhances readability and maintains a consistent visual structure.
● Braces: Place opening braces on the same line as the declaration. For example:
● java
● Copy code
3. Comments and Documentation
● Javadoc: Use Javadoc comments for classes, methods, and fields to generate API documentation. This improves code documentation and helps developers understand the purpose of each component.
● Inline Comments: Add comments sparingly to clarify complex logic or to provide context where necessary. Avoid redundant or obvious comments.
4. Exception Handling
● Catch Specific Exceptions: Catch specific exceptions rather than using a generic Exception catch-all block. This promotes better error handling and debugging.
● Throw Early, Catch Late: If a method cannot handle an exception, let it propagate up the call stack. Only catch exceptions where you can take appropriate action.
5. Code Organization
● Package Structure: Organize classes into logical packages based on functionality. This helps in maintaining a clear project structure and avoids clutter.
● Single Responsibility Principle: Each class should have a single responsibility, adhering to the SOLID principles. This enhances modularity and makes the codebase easier to understand and modify.
6. Use of Constants
● Avoid Magic Numbers: Define constants for frequently used values to improve code readability and maintenance. For example:
7. Testing Guidelines
● Unit Tests: Write unit tests for each class or method to ensure its correctness and to facilitate refactoring.
● Test-Driven Development (TDD): Consider adopting TDD practices, where tests are written before the actual code. This encourages a more robust and testable design.
Conclusion
In the world of Java development, adhering to coding standards is not just a best practice—it's a necessity. These guidelines serve as a roadmap for developers, guiding them towards writing cleaner, more maintainable, and bug-free code.
By prioritizing clarity, consistency, and code quality, teams can create software that not only functions flawlessly but is also a joy to work with. So, the next time you embark on a Java coding